What is Java?
Java is a popular programming language, created in 1995.
It is owned by Oracle, and more than 3 billion devices run Java.
It is used for:
- Mobile applications (specially Android apps)
- Desktop applications
- Web applications
- Web servers and application servers
- Games
- Database connection
- And much, much more!
Java - What is OOP?
OOP stands for Object-Oriented Programming.
Procedural programming is about writing procedures or methods that perform operations on the data, while object-oriented programming is about creating objects that contain both data and methods.
Object-oriented programming has several advantages over procedural programming:
- OOP is faster and easier to execute
- OOP provides a clear structure for the programs
- OOP helps to keep the Java code DRY "Don't Repeat Yourself", and makes the code easier to maintain, modify and debug
- OOP makes it possible to create full reusable applications with less code and shorter development time
Tip: The "Don't Repeat Yourself" (DRY) principle is about reducing the repetition of code. You should extract out the codes that are common for the application, and place them at a single place and reuse them instead of repeating it.
Java - What are Classes and Objects?
Classes and objects are the two main aspects of object-oriented programming.
Look at the following illustration to see the difference between class and objects:
class
Fruit
objects
Apple
Banana
Mango
Another example:
class
Car
objects
Volvo
Audi
Toyota
So, a class is a template for objects, and an object is an instance of a class.
When the individual objects are created, they inherit all the variables and methods from the class.
Java Syntax
In the previous chapter, we created a Java file called Main.java
, and we used the following code to print "Hello World" to the screen:
Main.java
public class Main {
public static void main(String[] args) {
System.out.println("Hello World");
}
}
Example explained
Every line of code that runs in Java must be inside a class
. In our example, we named the class Main. A class should always start with an uppercase first letter.
Note: Java is case-sensitive: "MyClass" and "myclass" has different meaning.
The name of the java file must match the class name. When saving the file, save it using the class name and add ".java" to the end of the filename. To run the example above on your computer, make sure that Java is properly installed: Go to the Get Started Chapter for how to install Java. The output should be:
Hello World
The main Method
The main()
method is required and you will see it in every Java program:
public static void main(String[] args)
Any code inside the main()
method will be executed. Don't worry about the keywords before and after main. You will get to know them bit by bit while reading this tutorial.
For now, just remember that every Java program has a class
name which must match the filename, and that every program must contain the main()
method.
System.out.println()
Inside the main()
method, we can use the println()
method to print a line of text to the screen:
public static void main(String[] args) {
System.out.println("Hello World");
}
Note: The curly braces {}
marks the beginning and the end of a block of code.
System
is a built-in Java class that contains useful members, such as out
, which is short for "output". The println()
method, short for "print line", is used to print a value to the screen (or a file).
Don't worry too much about System
, out
and println()
. Just know that you need them together to print stuff to the screen.
You should also note that each code statement must end with a semicolon (;
).
System.out.println() liste n:
public class listed line {
public static void main(String args[])
{
int numbers[]={10,20,30,40,50};
for(int n : numbers)
{
System.out.println(n);
}
}
}
Output:
Java Control Statements | Control Flow in Java
Java compiler executes the code from top to bottom. The statements in the code are executed according to the order in which they appear. However, Java provides statements that can be used to control the flow of Java code. Such statements are called control flow statements. It is one of the fundamental features of Java, which provides a smooth flow of program.
Java provides three types of control flow statements.
- Decision Making statements
- if statements
- switch statement
- Loop statements
- do while loop
- while loop
- for loop
- for-each loop
- Jump statements
- break statement
- continue statement
Loop Statements
In programming, sometimes we need to execute the block of code repeatedly while some condition evaluates to true. However, loop statements are used to execute the set of instructions in a repeated order. The execution of the set of instructions depends upon a particular condition.
In Java, we have three types of loops that execute similarly. However, there are differences in their syntax and condition checking time.
- for loop
- while loop
- do-while loop
Let's understand the loop statements one by one.
Java for loop
In Java, for loop is similar to C and C++. It enables us to initialize the loop variable, check the condition, and increment/decrement in a single line of code. We use the for loop only when we exactly know the number of times, we want to execute the block of code.
The flow chart for the for-loop is given below.
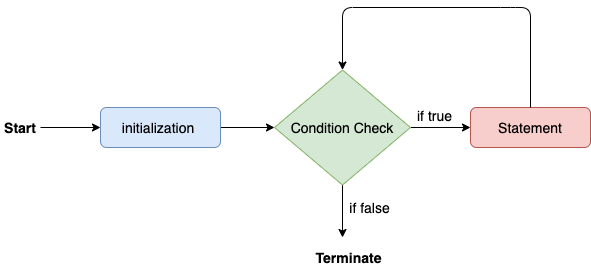
Consider the following example to understand the proper functioning of the for loop in java.
Calculation.java
Output:
The sum of first 10 natural numbers is 55
Java for-each loop
Java provides an enhanced for loop to traverse the data structures like array or collection. In the for-each loop, we don't need to update the loop variable. The syntax to use the for-each loop in java is given below.
Consider the following example to understand the functioning of the for-each loop in Java.
Calculation.java
Output:
Printing the content of the array names: Java C C++ Python JavaScript
Java while loop
The while loop is also used to iterate over the number of statements multiple times. However, if we don't know the number of iterations in advance, it is recommended to use a while loop. Unlike for loop, the initialization and increment/decrement doesn't take place inside the loop statement in while loop.
It is also known as the entry-controlled loop since the condition is checked at the start of the loop. If the condition is true, then the loop body will be executed; otherwise, the statements after the loop will be executed.
The syntax of the while loop is given below.
The flow chart for the while loop is given in the following image.
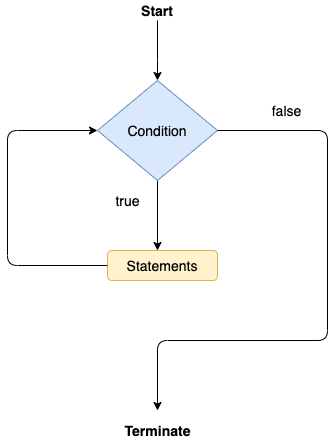
Consider the following example.
Calculation .java
Output:
Printing the list of first 10 even numbers 0 2 4 6 8 10
Java do-while loop
The do-while loop checks the condition at the end of the loop after executing the loop statements. When the number of iteration is not known and we have to execute the loop at least once, we can use do-while loop.
It is also known as the exit-controlled loop since the condition is not checked in advance. The syntax of the do-while loop is given below.
The flow chart of the do-while loop is given in the following image.
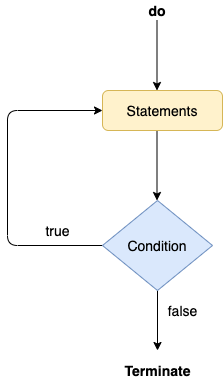
Consider the following example to understand the functioning of the do-while loop in Java.
Calculation.java
Output:
Printing the list of first 10 even numbers 0 2 4 6 8 10
Jump Statements
Jump statements are used to transfer the control of the program to the specific statements. In other words, jump statements transfer the execution control to the other part of the program. There are two types of jump statements in Java, i.e., break and continue.
Java break statement
As the name suggests, the break statement is used to break the current flow of the program and transfer the control to the next statement outside a loop or switch statement. However, it breaks only the inner loop in the case of the nested loop.
The break statement cannot be used independently in the Java program, i.e., it can only be written inside the loop or switch statement.
The break statement example with for loop
Consider the following example in which we have used the break statement with the for loop.
BreakExample.java
Output:
0 1 2 3 4 5 6
break statement example with labeled for loop
Calculation.java
Output:
0 1 2 3 4 5
Java continue statement
Unlike break statement, the continue statement doesn't break the loop, whereas, it skips the specific part of the loop and jumps to the next iteration of the loop immediately.
Consider the following example to understand the functioning of the continue statement in Java.
Output:
0 1 2 3 5 1 2 3 5 2 3 5